Rule engines have become an essential component in modern software systems, enabling developers to separate business logic from application code for greater flexibility and maintainability. In the context of C#, rule engines are particularly powerful, allowing for dynamic decision-making processes in a wide range of applications, from financial systems to e-commerce platforms.
A rule engine in C# evaluates business rules defined by conditions and actions, ensuring that applications remain adaptable to changing requirements without extensive code changes. Whether you're looking for a simple rules engine for lightweight tasks or an open-source solution for complex workflows, C# offers a rich ecosystem of tools and libraries to cater to different needs.
In this blog, we’ll explore how to implement a rule engine in C#, complete with examples and use cases. From building a simple accounting rules engine to leveraging open-source solutions, we’ll guide you through the process of creating and managing dynamic business rules efficiently. If you’re seeking to streamline your logic management and improve scalability, this guide will provide the insights you need to get started.
Overview of C# Business Rules Engines
A Business Rules Engine (BRE) in C# is a software component that separates business logic from the core application code, enabling dynamic rule management and reducing the need for extensive coding during updates. This modular approach ensures that business logic remains flexible, maintainable, and scalable, aligning with rapidly changing requirements across various industries.
How C# Business Rules Engines Function?
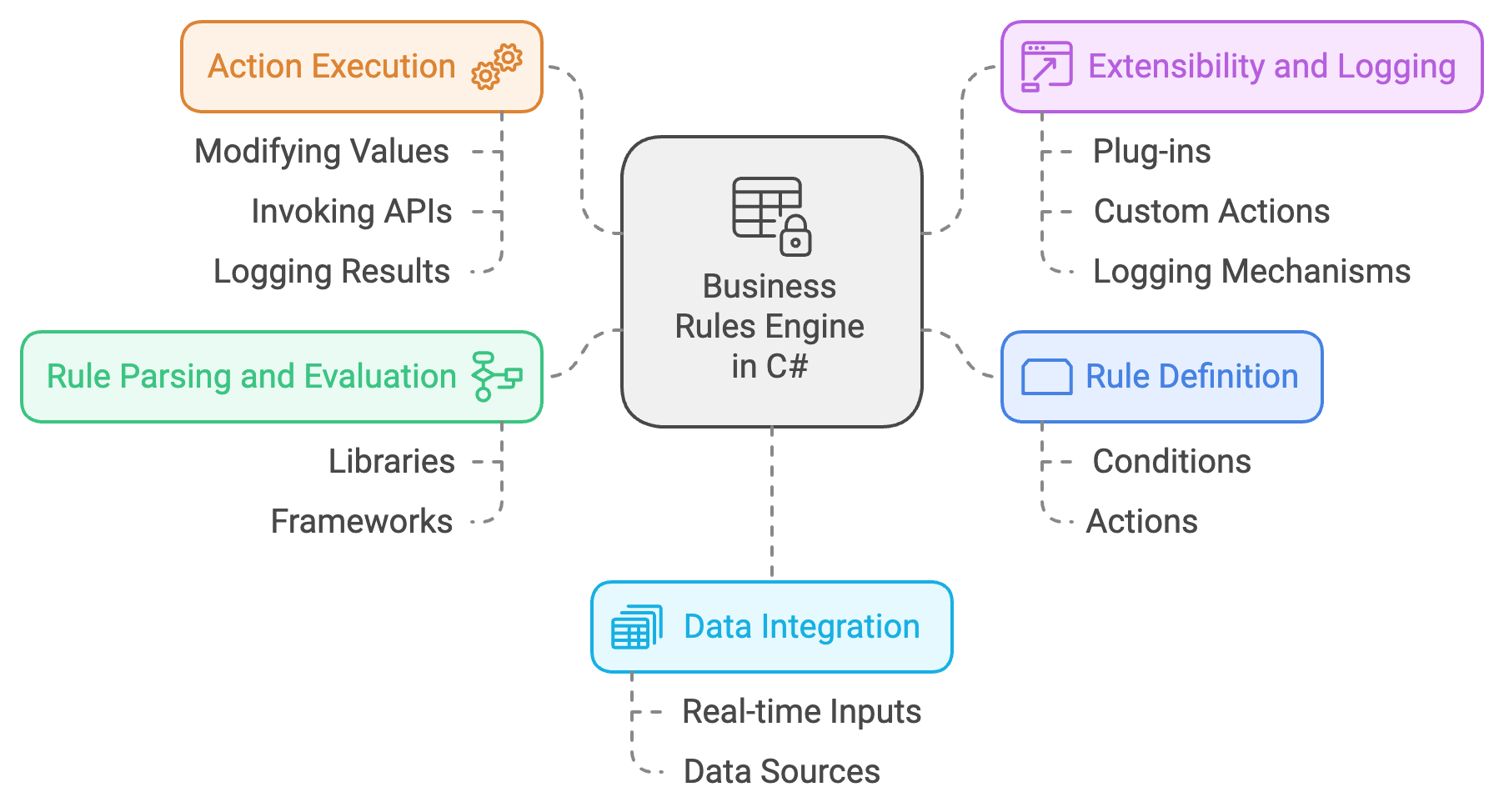
In the C# ecosystem, a Business Rules Engine typically functions as a decision-making system that evaluates conditions and triggers actions based on predefined rules. Here’s an overview of the process:
- Rule Definition:
- Business rules are defined as conditions and actions, often in a declarative or table-based format. For example:
- Condition: If
order_total > 500
andcustomer_type == "premium"
. - Action: Apply a 10% discount.
- Condition: If
- Rule Parsing and Evaluation:
- The BRE parses these rules and evaluates them against runtime data, such as user input or database values. C# provides robust libraries and frameworks, such as RulesEngine.NET or NRules, to facilitate this process.
- Data Integration:
- Rule engines seamlessly integrate with data sources, fetching real-time inputs like inventory levels, customer data, or transaction history to evaluate rules dynamically.
- Action Execution:
- Based on the rule evaluation, the engine triggers actions such as modifying a value, invoking APIs, or logging results. For instance, a C# rule engine might update a database entry or send a notification.
- Extensibility and Logging:
- Advanced BREs in C# support extensible logic through plug-ins, custom actions, and logging mechanisms to track rule execution and outcomes.
Common Features of C# Rule Engines
- Declarative Rule Definition: Enables business users or developers to define rules in a human-readable format.
- Dynamic Rule Management: Allows real-time updates without recompiling the application.
- Priority and Conflict Resolution: Handles overlapping or conflicting rules by assigning priorities.
- Integration: Seamlessly integrates with APIs, databases, and other services.
- Scalability: Supports complex decision-making logic across large datasets or high transaction volumes.
Advantages of Using C# for Business Rules Engines
- Robust Libraries: The C# ecosystem includes powerful BRE frameworks such as NRules, RulesEngine.NET, and Drools.NET, making it easier to implement rule-based systems.
- Integration Capabilities: C# seamlessly integrates with enterprise systems like CRM, ERP, and databases, ensuring smooth data flow.
- Performance: Optimized for high performance and scalability, suitable for large-scale applications.
- Strong Typing: Ensures safer rule definitions and reduces runtime errors.
C# rule engines bring unparalleled flexibility and efficiency to rule-based processing, making them invaluable across industries. In the next section, we’ll dive into practical examples of implementing a C# rule engine, providing actionable insights and code snippets for developers to get started.
Also read: Python Rule Engines
How to Create a Rule Using C#?
Creating a complex rule in C# involves defining conditions, integrating dynamic data, and setting up actions to execute based on the rule’s evaluation. With powerful frameworks like NRules or RulesEngine.NET, C# makes it easier to design and manage complex rules. Let’s walk through the steps to create a robust rule engine capable of handling intricate logic.
Step 1: Choose a C# Rule Engine Framework
Frameworks like NRules or RulesEngine.NET simplify rule creation and evaluation. These libraries provide declarative syntax and tools for dynamic rule management. In this example, we’ll use RulesEngine.NET to demonstrate building a complex rule.
Step 2: Define the Rule Logic
Complex rules often involve multiple conditions and actions. For instance:
- Condition: If
order_total > 500
,customer_type == "premium"
, andinventory_status == "low"
. - Action: Apply a 15% discount and notify the inventory manager.
RulesEngine.NET supports defining such rules in a JSON format for easier management.
Example JSON Rule:
[
{
"WorkflowName": "DiscountWorkflow",
"Rules": [
{
"RuleName": "HighValuePremiumCustomer",
"SuccessEvent": "ApplyDiscount",
"ErrorMessage": "Rule condition not met",
"Conditions": {
"All": [
{
"Name": "OrderTotal",
"Operator": ">",
"Value": 500
},
{
"Name": "CustomerType",
"Operator": "==",
"Value": "premium"
},
{
"Name": "InventoryStatus",
"Operator": "==",
"Value": "low"
}
]
}
}
]
}
]
Step 3: Set Up the Data Context
Rules require runtime data for evaluation. Prepare a context class that represents the data inputs for your rule engine.
Example Context Class:
public class OrderContext
{
public decimal OrderTotal { get; set; }
public string CustomerType { get; set; }
public string InventoryStatus { get; set; }
}
Step 4: Load and Evaluate Rules
Load the JSON rules into the rule engine and evaluate them against the provided data.
using RulesEngine;
using System;
using System.Collections.Generic;
class Program
{
static void Main(string[] args)
{
// Define rule in JSON format
string jsonRules = System.IO.File.ReadAllText("rules.json");
// Initialize the RulesEngine
var workflows = Newtonsoft.Json.JsonConvert.DeserializeObject<Workflow[]>(jsonRules);
var rulesEngine = new RulesEngine.RulesEngine(workflows, null);
// Prepare data context
var orderContext = new OrderContext
{
OrderTotal = 600,
CustomerType = "premium",
InventoryStatus = "low"
};
var inputs = new[] { new RuleParameter("order", orderContext) };
// Evaluate the rule
var result = rulesEngine.ExecuteAllRulesAsync("DiscountWorkflow", inputs).Result;
// Handle the result
foreach (var res in result)
{
if (res.IsSuccess)
{
Console.WriteLine($"Success: {res.Rule.RuleName} - {res.SuccessEvent}");
}
else
{
Console.WriteLine($"Failed: {res.Rule.RuleName} - {res.ErrorMessage}");
}
}
}
}
Step 5: Add Actions for Successful Rule Evaluation
Actions triggered by rule evaluation can include API calls, database updates, or sending notifications.
if (result.IsSuccess)
{
// Apply discount
Console.WriteLine("15% discount applied.");
// Notify inventory manager
Console.WriteLine("Inventory manager notified about low stock.");
}
Step 6: Test and Optimize
Before deploying the rule engine, test it under various scenarios to ensure it handles all edge cases.
- Use unit tests to validate each condition and action.
- Optimize the rules for performance by reducing redundant conditions or grouping similar logic.
By following these steps, you can create a powerful rule engine in C# capable of handling complex logic with minimal effort. This approach ensures flexibility, scalability, and maintainability, making it ideal for modern business needs.
Also read: SQL Rule Engine
Challenges of Using C# Rule Engine
While C# rule engines offer significant flexibility and power, they come with challenges that can hinder implementation and long-term management. Below are some of the key problems and how tools like Nected can address them effortlessly.
1. Complex Rule Management
As business requirements grow, managing a large set of rules becomes increasingly complex. Rules are often hardcoded or stored in JSON files, making it challenging to update, debug, or add new rules without risking errors or inconsistencies.
Nected provides a visual Decision Table editor that allows users to define, organize, and update rules in a structured and human-readable format. Non-technical users can modify rules without writing or editing code, reducing the risk of errors and making rule management far more efficient.
2. High Development and Maintenance Overhead
Developing a C# rule engine from scratch requires significant coding, testing, and debugging. Additionally, maintaining the engine as business rules evolve demands continuous developer involvement, leading to high resource consumption.
Nected's low-code platform eliminates the need for extensive coding. With its drag-and-drop interface and pre-built workflows, businesses can implement and maintain complex dotnet rule engines without heavy reliance on developers. This reduces development costs and accelerates time-to-market by up to 70%.
3. Difficulty in Integrating with Real-Time Data Sources
Rule engines often require integration with multiple data sources, such as databases, APIs, or external services, to evaluate rules dynamically. Setting up and maintaining these integrations in C# can be complex and time-consuming.
Nected offers seamless API and database integrations, enabling users to fetch real-time data effortlessly. Inputs like order_total
, customer_type
, or inventory_status
can be directly mapped to rules without writing custom integration code.
4. Scalability Issues
As the number of rules and datasets grows, C# rule engines may face performance bottlenecks. Scaling these engines to handle complex, high-volume scenarios often requires reengineering the system.
Nected is designed for scalability, allowing businesses to manage thousands of rules across multiple datasets with ease. Features like grouped conditions and parallel workflows ensure efficient performance even in large-scale environments.
5. Dependency on Technical Expertise
C# rule engines typically require skilled developers for implementation, updates, and troubleshooting. This dependency creates bottlenecks, especially when rapid iterations or new features are needed.
Nected empowers non-technical users to manage and update rules independently through its intuitive interface. Teams can focus on strategic tasks rather than technical implementation, reducing bottlenecks and enhancing agility.
6. Error-Prone Rule Evaluation
Manually coding rules or writing JSON can lead to syntax errors, logical inconsistencies, or overlooked conditions, which can cause incorrect rule evaluation.
Nected validates rules automatically as they are created or updated, ensuring they are error-free before execution. This reduces debugging time and prevents costly mistakes in rule evaluation.
While C# rule engines are powerful, they come with challenges that can complicate implementation and management. Tools like Nected address these issues with a low-code approach, visual tools, seamless integrations, and built-in analytics. By leveraging Nected, businesses can build and manage rule engines more efficiently, reducing costs, accelerating deployment, and improving scalability.
How to Build C#-Level Rules Using Nected?
Building complex rules that rival the flexibility and logic of a C# rule engine is straightforward with Nected’s intuitive platform. Nected provides visual tools like Decision Tables and workflows, enabling businesses to create and manage dynamic rules without extensive coding. Here’s a step-by-step guide to building C#-level complex rules using Nected.
Step 1: Create a New Decision Table
Start by creating a Decision Table, which will serve as the foundation for your rules. Access the Nected dashboard, navigate to the Decision Table section, and create a new table. Name it appropriately, such as "Discount Workflow Rules."
Step 2: Add Input Attributes
Define the inputs your rule will use for evaluation. These are equivalent to the variables in a C# rule engine. Add attributes such as:
order_total
customer_type
inventory_status
You can also integrate these inputs dynamically from APIs or databases if required.
Step 3: Define Conditions and Actions
Set up the conditions and corresponding actions for your rules in the Decision Table. For example:
- Condition:
order_total > 500
andcustomer_type == "premium"
andinventory_status == "low"
- Action: Apply a 15% discount and trigger a notification.
Example Decision Table:
ConditionActionorder_total > 500
AND customer_type == "premium"
AND inventory_status == "low"
Apply a 15% discount; Notify inventory team.order_total < 100
Offer free shipping.
Step 4: Use Custom Formulas for Complex Calculations
For dynamic calculations, use Nected’s formula builder. For example, calculate a dynamic discount based on order value:
- Formula:
discount = order_total * 0.15
These formulas eliminate the need for manual coding and can be reused across multiple rules.
Step 5: Integrate Real-Time Data Sources
Connect real-time data sources such as APIs, or databases to fetch input values dynamically. For example, use an API to get inventory status or customer type, ensuring your rules are always based on the latest data.
Step 6: Test and Validate the Rules
Run simulations in Nected’s testing environment to ensure your rules behave as expected under various scenarios. For example, test inputs with different order_total
values to verify discount application.
Step 7: Optional - Add Workflows for Advanced Flow
If you need additional actions beyond rule evaluation, like sending notifications or updating systems, use Nected’s workflow editor. For instance:
- If
inventory_status == "low"
, send an email to the inventory team. - If
order_total > 500
, update the CRM with customer details.
Step 8: Deploy and Monitor
Once validated, deploy the rules directly to your application. Use Nected’s analytics tools to monitor rule performance and optimize them as needed.
Here’s why Nected Works better for Complex rules:
- Simplicity: Visual Decision Tables replace the need for complex JSON or code.
- Dynamic Data Integration: Fetch inputs in real-time to ensure accurate rule evaluation.
- Scalability: Easily add or modify rules without breaking existing logic.
- Error Prevention: Built-in validation ensures rules are error-free before deployment.
With Nected, you can replicate and enhance the functionality of a C# rule engine, all while saving development time and reducing maintenance complexity. This approach is ideal for businesses looking to scale quickly while maintaining robust logic management.
Conclusion
C# rule engines are powerful tools for implementing dynamic and flexible business logic. However, they often come with challenges such as high development overhead, complex integrations, and scalability issues. Tools like Nected provide an intuitive and efficient alternative, enabling businesses to build and manage complex rules without extensive coding. By leveraging visual Decision Tables, real-time data integrations, and workflows, Nected empowers users to achieve the same functionality as C# rule engines while reducing costs and accelerating deployment. Whether you’re managing pricing, customer segmentation, or operational logic, Nected provides a scalable, low-code solution tailored to modern business needs.
FAQs
1. What is a C# rule engine, and why is it important?
A C# rule engine is a software component that evaluates business rules based on conditions and actions. It helps separate business logic from core application code, making applications more flexible and maintainable. It’s commonly used in scenarios like dynamic pricing, fraud detection, and workflow automation.
2. How does Nected compare to a C# rule engine?
While C# rule engines require significant coding and maintenance, Nected offers a low-code platform that simplifies rule creation and management. It provides visual tools, real-time data integration, and built-in workflows, enabling users to build complex rules without the need for extensive technical expertise.
3. Can Nected handle complex, nested logic like a C# rule engine?
Yes, Nected supports complex, nested conditions through its grouped conditions feature in Decision Tables. You can define intricate logic visually, making it as powerful as a C# rule engine while being easier to manage.
4. What industries can benefit from using Nected for rule management?
Nected is versatile and can be used across industries such as e-commerce (dynamic pricing, promotions), finance (credit scoring, fraud detection), healthcare (claims validation), and logistics (route optimization, inventory management).
5. How secure is Nected when handling sensitive business rules and data?
Nected follows industry-standard security practices, including data encryption, role-based access control, and secure API integrations. This ensures your business rules and data are managed securely and reliably.