By definition, a Rule engine is a software system that executes one or more rules in a runtime production environment. Jess (Java Expert System Shell) is one of the earliest rule engines in Java that can be integrated. Jess uses an enhanced implementation of the highly efficient Rete algorithm, making it much faster than a simple Java loop for most scenarios. Jess is like the CLIPS expert system, which means it's referring to a rule engine for the Java platform. Jess programming language is based on LISP-like syntax, it processes and infers data using rules. LISP (LISt Processing) is one of the oldest high-level programming languages, designed for symbolic computation and known for its distinctive use of parenthesized prefix notation. A rule engine, specifically in the case of Jess, parses through a set of rules and applies them to data by modularizing decision-making (by evaluating conditions specified within said rules), content process automation (executing actions from inside these pieces) etc.
Key features:-
- Sophisticated rule engine capable of executing complex rules and logical expressions efficiently.
- Tight integration with Java, allowing for the direct manipulation of Java objects and leveraging existing Java libraries.
- Forward and backward chaining support, enabling versatile problem-solving mechanisms.
- A scripting environment that supports interactive development and testing of rules.
- Extensive API for programmatically managing rules and integrating Jess with other applications.
In this blog, we will talk about the features of Jess, the challenges associated with using it, and the solutions available to overcome these challenges.
Implementing Custom Rule Engines with JESS
Rules and facts can be created and managed using a custom rule engine developed based on Jess. These rules allow for data processing and decision-making based on specific conditions. Rules can be executed from rule sets written in the native Jess Rules Language or from a more verbose XML format. For simplicity, the native format will be used.
Here's a simple example to illustrate rule implementation in Jess. This example demonstrates basic rule creation and fact assertion to determine if a person is eligible to vote based on their age. We’ll save the rules file as VotingEligibility.clp.
Explanation:
- Templates: We define two templates
- person with slots for name and age.
- eligible with a slot for name.
- Rules:
- check-eligibility: This rule fires if a person's age is 18 or older. It asserts an eligible fact and prints that the person is eligible to vote.
- not-eligible: This rule fires if a person's age is less than 18. It prints that the person is not eligible to vote.
- Facts:
- The facts about people and their ages are asserted. For example, John is 20, Alice is 17, Bob is 18, and Carol is 16.
- Execution:
- The reset command initializes the rule engine.
- The assert commands add the facts to the working memory.
- The run command executes the rules based on the asserted facts.
Now, let’s create an instance of the Jess Rete rule engine, reset() it to its initial state, load up the rules in VotingEligibility.clp, and run them:
When we run our program :-
- The Jess rule engine (Rete object) is created and initialized.
- The VotingEligibility.clp file is loaded into the Jess rule engine using the batch method.
- The run method is called on the Jess engine, which processes the rules and facts loaded from the CLP file.
- The facts defined in the VotingEligibility.clp file are asserted.
- Based on the evaluation, the rules will trigger and print the following output to the console
John is eligible to vote.
Alice is not eligible to vote.
Bob is eligible to vote.
Carol is not eligible to vote.
Suggested read: Salesforce Business Rule Engine: Optimizing Financial Operations
Challenges and Limitations in JESS Rule Engine Development
Several challenges and limitations may arrive in the process of implementing a rule engine like Jess. All of this can impact the performance, maintainability and effectiveness from our rule engine.
- Interoperability: Jess Rule Engine is limited to Java environments, posing interoperability challenges for systems using other programming languages like .NET or Python. This constraint requires developers to learn Java, potentially increasing the learning curve and development time. Deployment issues may arise when running on non-Java platforms, restricting Jess's adoption outside the Java ecosystem.
- Complex Syntax: Developers with no experience in LISP, however will have to get over a steep learning curve, and will often receive errors on rule definitions because the syntax of rules is very specific.
- Performance Bottlenecks: Large sets of rules can have performance implications as all the rules must be checked against the facts by the rule engine. This may then decrease the time of processing, more so in real-time applications.
- Debugging and Testing: Debugging rules in the rule engine may be more difficult than traditional programming. Rules are abstract and do not directly contribute to data in a way which makes them hard to debug.
- Scalability: As the number of rules and facts increases, the rule engine may face scalability issues. Managing and maintaining a large set of rules can become cumbersome, requiring efficient rule management and optimization strategies.
- Limited Community Support: Compared to more popular rule engines, Jess has a smaller user community, which can make it harder to find resources, tutorials, and community support when facing issues or looking for best practices.
Addressing these challenges often requires careful planning, optimization, and sometimes choosing the right tools and frameworks that better align with the project’s needs.
Rule Engine Integration: Jess vs. Nected
Jess stands as a potent tool for Java developers needing to incorporate complex rule-based logic and AI into their applications, offering deep integration with Java and advanced reasoning capabilities. However, Nected offers a more accessible and cost-effective solution, particularly appealing to projects with limited technical resources or those seeking to reduce development and maintenance overhead. Nected's low/no-code approach, combined with its comprehensive support and scalability, presents a compelling alternative for a wide range of applications, emphasizing ease of use and efficient rule management.
Implementation through Nected:
Start by creating a new rule. You can either build from scratch or use one of our predefined templates to save time. Our user-friendly interface guides you through the process, ensuring a seamless experience.
- From Scratch: Ideal for custom rules tailored to your specific needs.
- From Predefined Templates: Quickly get started with our ready-made templates for common use cases.
Configure Rule Conditions:-
- Define Data Sources: Add data sources for your rule, which can be direct input attributes, datasets from your database, or data fetched from an external API.
- Set Input Attributes: If needed, you can add custom input attributes by clicking "Add Input Attributes" and defining the required fields.
- Create Conditions: Use the no-code editor to create rule conditions in the format: if -> attribute -> operator -> value. You can use various operators and logical combinations to define complex conditions.
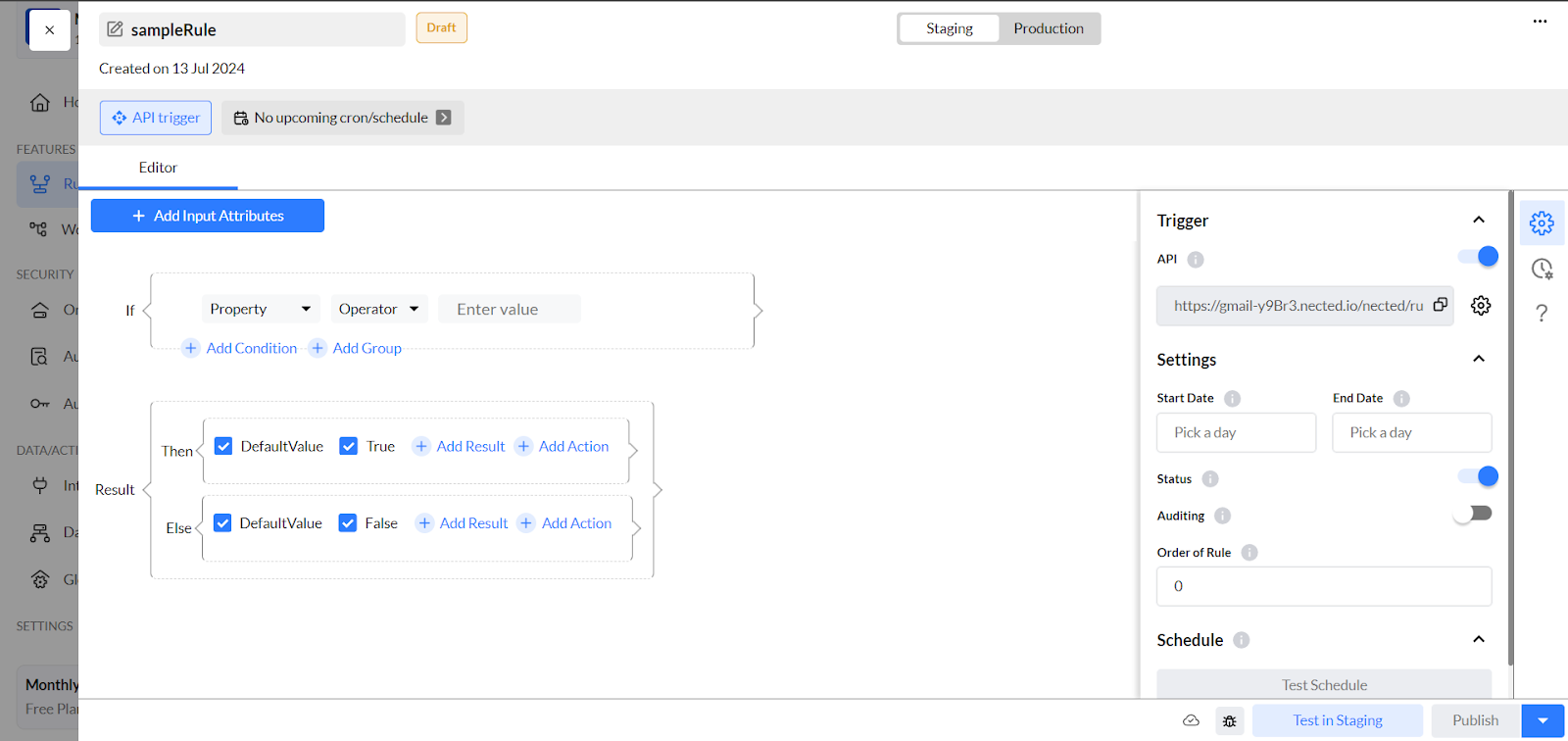
Define Actions: Specify what action the rule should take based on the result. This could involve updating a result or triggering a flow within your system or third-party systems via API connectors.
In summary, Nected emerges as a versatile and accessible solution for businesses seeking efficient rule management and decision-making capabilities. While other rule engines like Drools, Jess, and jBPM excel in specific technical domains or enterprise-level complexities, Nected's user-friendly low/no-code platform, advanced UI, seamless integration, and cost-effectiveness make it a compelling choice for a wide range of applications. By reducing barriers to entry and enhancing usability without compromising on functionality, Nected simplifies rule deployment and management, empowering both technical and non-technical users to streamline their business processes effectively.
Also read our blog on Nected Rule Engine
Best Practices for Building a Rule Engine on Jess
To build a robust and efficient engine, Jess requires adhering best practices in safety, performance and scalability. So here are some key best practices:
- Modular Rule Design in Jess: Separate and group your Jess rules. Base your Jess rules on performance, group them into modules. This approach to modularity makes it easier for you to manage and administer the rule base. Utilize Jess templates for facts and rules to define common structures, this makes use of reusing elements and prevents one entity from being unnecessarily created. As a result, both maintainability is enhanced and readability maximized.
- Efficient Fact Management in Jess: In Jess, managing the working memory efficiently is crucial. Minimize the number of facts in the working memory by periodically removing obsolete or irrelevant facts. Use the retract function in Jess to dispose of facts that are no longer needed, keeping the working memory lightweight and responsive.
- Incremental Rule Testing with Jess: During development, incrementally test your Jess rules to catch issues early. Write unit tests for individual rules using Jess’s testing capabilities to validate correctness against expected outcomes. Use the (watch rules) command to display the rule that was triggered and help you figure out any problems in its approach.
- Optimization Techniques for Jess Rule Execution: Optimize rule execution in Jess by utilizing indexing and efficient pattern matching. To avoid needless evaluations, make sure the conditions are unique and selective. Use the (agenda-focus) and (focus) commands on your Jess rule engine across various workloads to profile and benchmark and avoid wide area performance bottlenecks.
- Documentation and Commenting in Jess: Document thoroughly each Jess rule, including the underlying reasoning as well as the intent. In your Jess rules use comments to detail complex logic and any suppositions. This allows other programmers to understand what is meant by the rules, and makes things smoother for maintenance or enhancement.
- Version Control and Change Management: Use version control systems to track changes to rules and templates. This helps in maintaining a history of modifications and rolling back if needed. Implement a change management process to review and approve changes to the rule base, ensuring that modifications are well-considered and tested.
Nected follows these best practices while implementing rule engines. By using Nected, you can leverage a well-designed, optimized, and maintainable rule engine without the hassle of managing these complexities in-house.
Sign up now to experience the benefits of a professionally managed rule engine solution.
Conclusion
In summary, Jess is one powerful weapon that Java programmers can wield when it comes to building complex rule-based systems and AI into your app. Jess is well suited to such situations as Jess provides a rich Java Integration for the depth of decision-making complexity along with superb Reasoning capabilities.
On the other hand, in a technology landscape that is changing quickly, where availability and being user-friendly are key. Things are slowly changing with tools like Nected which make the experience way easier by moving to a higher level of abstraction and providing no/low-code platform. Not only does Nected make rule management simpler, but also facilitates seamless collaboration with non-technical team members and technical teams in all levels of proficiency -making it perfect for projects beloved by those guidelines.
Organizations interested in simplifying rule deployment and operations efficiency have another option courtesy of Nected, which touts usability and scalability. Ultimately, the choice between Jess and Nected depends on specific project requirements, technical capabilities, and the desired balance between advanced functionality and ease of implementation.
As businesses navigate the complexities of rule-based systems, exploring tools like Jess and Nected provides invaluable insights into optimizing decision processes and enhancing business outcomes through innovative technology solutions.
Jess Rule Engine FAQs
Q1: What is Jess, and how does it work?
Jess (Java Expert System Shell) is a Java-based rule engine and scripting environment used for building rule-based systems that execute actions based on defined rules and facts.
Q2: Can Jess integrate with other Java applications?
Yes, Jess can be embedded within Java applications, allowing direct interaction between Jess rules and Java objects.
Q3: Why should I consider using Nected for my rule engine needs?
Nected is a clean, fast and maintainable rule engine manager. It enforces best practices, provides better scalability and performance, and simplifies the rule engine implementation on your system.
Q4: What are the advantages of using Nected over Jess or other rule engines?
Nected provides a simpler user interface and broader integration capabilities, making it easier to create rule engines without in-depth technical understanding. This is for improved performance, scalability and support reducing the complexity and overhead of having an in-house version.